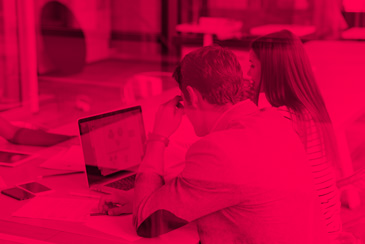
Uploading and Minting NFTs with Pinata and ERC721 on a Next.js NFT Marketplace
In this blog post, we will explore the process of uploading images using Pinata, a decentralized file-sharing platform, and minting Non-Fungible Tokens (NFTs) using the ERC721 standard on a Next.js NFT marketplace. By integrating Pinata and ERC721, we can create a seamless flow for users to upload artwork, generate unique NFTs, and showcase them on our marketplace. We will delve into the setup and installation process, discuss the workflow for uploading images to Pinata, explore the implementation of ERC721 for minting NFTs, and demonstrate how to present them on our Next.js NFT marketplace.
Setting up the Next.js NFT Marketplace
-
Start by setting up a Next.js project using the Next.js CLI or boilerplate templates. Next.js provides a robust foundation for building server-side rendered (SSR) React applications, aligning well with the needs of an NFT marketplace.
-
Install the necessary dependencies to interact with Ethereum and deploy smart contracts. Libraries like ethers.js or web3.js work well for this purpose. These libraries enable communication with the Ethereum network, allowing us to interact with smart contracts and mint NFTs.
-
Design and develop the UI for the NFT marketplace. Create pages for artwork listings, artists' profiles, and individual NFT details. Incorporate features like bidding, buying, and selling NFTs, considering the specific requirements and functionalities of the marketplace.
Uploading Images with Pinata
-
Register for a Pinata account and obtain your API keys. Pinata simplifies the process of storing and retrieving files in a decentralized manner using IPFS (InterPlanetary File System).
-
Install the pinata-sdk package to interact with Pinata programmatically. This SDK provides an easy-to-use interface for uploading files to Pinata and retrieving the corresponding file URLs required for minting NFTs.
-
Implement the image upload functionality in your Next.js application. Utilize the Pinata SDK to upload the images selected by users, using the Pinata API keys generated in the previous step.
-
Retrieve the file URLs returned by Pinata after successful uploads. These URLs will be used as references when minting the NFTs.
Implementing ERC721 for NFT Minting
-
Create an ERC721-compliant smart contract using tools like Truffle or Hardhat. ERC721 is a widely-used standard for building NFTs on Ethereum, ensuring compatibility across different marketplaces and wallets.
-
Define the required metadata for each NFT, including attributes like title, description, image URL, and any additional custom properties. These metadata attributes will provide essential information about the NFT and will be linked to the token through their respective token IDs.
-
Implement the minting functionality within the smart contract. This function allows users to mint new NFTs by providing the necessary metadata, such as the image URL obtained from Pinata. This function generates a unique token ID for each minted NFT, preserving its distinctiveness within the marketplace.
-
Integrate the smart contract into your Next.js application. Interact with the smart contract using libraries like ethers.js or web3.js to mint NFTs when triggered by users. Make sure to handle authentication and transaction management to ensure a secure and smooth minting process.
Showcasing NFTs on the Next.js Marketplace
-
Develop the necessary components and pages within your Next.js application to display NFTs. Implement a page to showcase individual NFT details, including the image, metadata, artist information, and any relevant bidding or buying options.
-
Fetch the metadata of the NFTs from the smart contract and display it accordingly on the marketplace. Utilize the metadata attributes stored during the minting process to present a detailed and captivating view of each NFT.
-
Integrate bidding and buying functionalities into the marketplace pages, allowing users to interact with NFTs by submitting bids or purchasing them. Connect these functionalities with the smart contract to ensure secure transactions and accurate updates to ownership records.
Conclusion
By combining the features of Pinata for image uploads and ERC721 for NFT minting, we have successfully created a seamless workflow for artists to upload artwork, generate unique NFTs, and showcase them on our Next.js NFT marketplace. This integration enables users to easily contribute their creations, ensuring a vibrant and diverse content collection within the marketplace. By utilizing the Pinata SDK and ERC721 standards, we have provided a user-friendly experience for artists and collectors alike, fostering engagement, transactions, and the growth of the NFT ecosystem. Remember to regularly update and refine your Next.js NFT marketplace based on user feedback and evolving industry trends. By continuously enhancing features such as bidding, buying, and selling functionalities, you can provide an immersive and dynamic experience for users, furthering the success and adoption of your NFT marketplace.