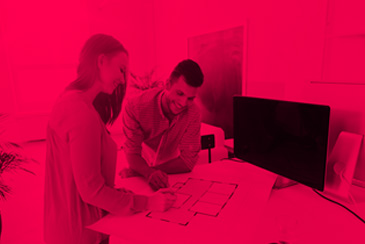
Building a Robust Backend with NestJS, TypeScript, and TypeORM for Your Next App
In the ever-evolving world of web development, creating a powerful backend for your application is crucial. NestJS, with TypeScript and TypeORM, offers a fantastic combination to build a robust and maintainable server-side component. In this blog, we’ll explore how to create a backend for your next app using these technologies, with PostgreSQL as our database of choice.
What is NestJS?
NestJS is a Node.js framework that provides a modular, extensible structure for building scalable and maintainable server-side applications. It’s built with TypeScript in mind, allowing for strong typing, improved developer tooling, and enhanced code quality. NestJS takes inspiration from Angular’s architecture, which makes it familiar to developers who have worked with Angular in the past.
Why TypeScript?
TypeScript is a statically-typed superset of JavaScript that enhances code quality, helps catch errors at compile-time, and improves developer productivity. When used in conjunction with NestJS, TypeScript enables you to define clear interfaces, data types, and catch issues before they reach production.
TypeORM: The ORM of Choice
TypeORM is a powerful Object-Relational Mapping (ORM) library for TypeScript and JavaScript. It simplifies database interactions by allowing developers to define models, relationships, and queries using TypeScript decorators. This simplifies the process of working with databases, and it integrates seamlessly with NestJS.
PostgreSQL: A Reliable Database
For this blog, we’ll use PostgreSQL as our database of choice. PostgreSQL is a robust, open-source relational database management system known for its reliability, extensibility, and excellent support for complex data types and queries.
Setting Up Your NestJS Project
To get started, make sure you have Node.js and npm installed on your machine. Then, use the following commands to create a new NestJS project:
# Install NestJS CLI globally
npm install -g @nestjs/cli
# Create a new NestJS project
nest new your-next-app
This will generate the basic project structure for your app.
Configuring PostgreSQL and TypeORM
Now, you need to set up PostgreSQL and TypeORM for your NestJS project. First, ensure you have PostgreSQL installed on your machine, and then create a new database for your project. Next, install the required dependencies:
# Install TypeORM and PostgreSQL driver
npm install typeorm pg
# Create a TypeORM configuration file (ormconfig.json)
Here’s a sample ormconfig.json
file:
{
"type": "postgres",
"host": "localhost",
"port": 5432,
"username": "your_username",
"password": "your_password",
"database": "your_database",
"synchronize": true,
"logging": true,
"entities": ["src/**/*.entity.ts"],
"migrations": ["src/migration/**/*.ts"],
"subscribers": ["src/subscriber/**/*.ts"],
"cli": {
"entitiesDir": "src/entity",
"migrationsDir": "src/migration",
"subscribersDir": "src/subscriber"
}
}
Modify the values in this configuration file to match your PostgreSQL setup.
Creating a Sample Entity
Let’s create a sample entity using TypeORM. In your NestJS project, generate an entity class:
nest generate resource your-resource
This command generates a controller and a service for your resource. Now, define your entity inside the src/your-resource/your-resource.entity.ts
file:
import { Entity, PrimaryGeneratedColumn, Column } from "typeorm";
@Entity("your_resource")
export class YourResource {
@PrimaryGeneratedColumn()
id: number;
@Column()
name: string;
}
Building the Service and Controller
In your resource’s service (src/your-resource/your-resource.service.ts
), implement CRUD operations and business logic for your entity. The controller (src/your-resource/your-resource.controller.ts
) handles incoming HTTP requests and uses the service to interact with the database.
Connecting Your Controller and Service
To connect your controller and service, use dependency injection. In the controller, import and inject your service:
import { YourResourceService } from "./your-resource.service";
@Controller("your-resource")
export class YourResourceController {
constructor(private readonly yourResourceService: YourResourceService) {}
// ...
}
Testing Your API
You can now run your NestJS application and start testing your API:
npm run start
Visit http://localhost:3000/your-resource
to interact with your resource using the provided endpoints.
NestJS, TypeScript, TypeORM, and PostgreSQL form a potent stack for building scalable and maintainable server-side applications. This combination allows you to create a reliable, well-structured backend for your next app, ensuring strong typing, efficient database interactions, and code maintainability. So, if you’re planning to build a backend for your next project, consider this powerful technology stack as a solid foundation. Happy coding!