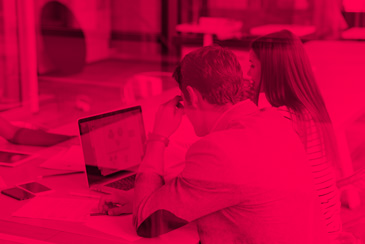
Supercharge Your React Native Application with Firebase and Algolia
In the fast-paced world of mobile app development, ensuring that your application’s search functionality is not only efficient but also user-friendly is a critical task. Firebase and Algolia offer a robust and scalable combination to build search functionality that enhances the user experience. In this blog, we’ll explore how you can integrate Firebase and Algolia into your React Native app for an exceptional search experience.
Firebase: The Real-time Database
Firebase is a comprehensive suite of tools that facilitates various app development tasks, from authentication and real-time database to cloud functions and analytics. For our discussion, we’ll focus on Firebase Realtime Database. This NoSQL, cloud-hosted database allows you to store and sync data across your mobile and web applications in real-time.
Algolia: The Search and Discovery Platform
Algolia is a powerful search and discovery API platform that simplifies the process of implementing advanced search functionality in your application. It offers lightning-fast search and browsing capabilities, ensuring that users can find what they’re looking for quickly.
Setting Up Firebase and React Native
To get started, make sure you have React Native and Node.js installed on your system. You can create a new React Native project using the following command:
npx react-native init YourApp
Next, set up Firebase by creating a project on the Firebase Console (https://console.firebase.google.com/). Follow the setup instructions, and make sure to enable the Firebase Realtime Database for your project.
Install Firebase in your React Native project:
npm install --save firebase
You’ll also need to install the necessary React Native Firebase packages, such as @react-native-firebase/app
, and configure them according to the documentation.
Firebase Realtime Database
With Firebase set up, you can start storing and retrieving data in real-time. Initialize Firebase in your app and access the Realtime Database:
import firebase from "firebase/app";
import "firebase/database";
const firebaseConfig = {
apiKey: "YOUR_API_KEY",
authDomain: "YOUR_AUTH_DOMAIN",
databaseURL: "YOUR_DATABASE_URL",
projectId: "YOUR_PROJECT_ID",
storageBucket: "YOUR_STORAGE_BUCKET",
messagingSenderId: "YOUR_MESSAGING_SENDER_ID",
appId: "YOUR_APP_ID",
};
if (!firebase.apps.length) {
firebase.initializeApp(firebaseConfig);
}
const database = firebase.database();
Now, you can start pushing and retrieving data from the Realtime Database.
Algolia Integration
To make your data searchable, you’ll need to index it in Algolia. Sign up for an Algolia account, create an index, and configure your data to sync with Algolia. You can use Algolia’s JavaScript client to perform indexing operations.
Here’s a basic example of pushing data to Algolia:
const algoliasearch = require("algoliasearch/lite");
const client = algoliasearch("YOUR_APP_ID", "YOUR_SEARCH_ONLY_API_KEY");
const index = client.initIndex("your_index_name");
const data = [
// Your data here
];
index.saveObjects(data).then(({ objectIDs }) => {
console.log("Indexed records with objectIDs:", objectIDs);
});
You can then use Algolia’s React InstantSearch library in your React Native app to implement search functionality easily. This library provides pre-built components for search interfaces, making it user-friendly and highly customizable.
Real-time Search with Algolia
One of the significant advantages of this setup is the real-time search experience. Algolia’s search results update instantly as new data is added or modified in the Firebase Realtime Database. This provides a seamless and responsive search experience for your app’s users.
Integrating Firebase and Algolia into your React Native app can supercharge your application’s search functionality. Firebase ensures that your data is stored and synced in real-time, while Algolia provides the powerful search and discovery capabilities that users demand. Combining these technologies will enable you to deliver a robust and user-friendly search experience that keeps your users engaged and satisfied. Happy coding!